mirror of
https://github.com/yanislav-igonin/micrach
synced 2024-12-22 06:12:33 +03:00
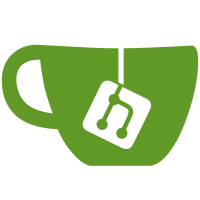
* feat: rename init migration * feat: add migration for is_archived field * fix: thread title wrap * fix: captcha checks after post validation now * fix * wip on migrate method * feat: decided to write own migrator * feat: add get files on folder func * wip * feat: add migrations table * feat: add migration method * doc * feat: query -> exec
41 lines
715 B
Go
41 lines
715 B
Go
package files
|
|
|
|
import (
|
|
"io/ioutil"
|
|
"log"
|
|
"os"
|
|
"path"
|
|
)
|
|
|
|
// Reads folder and returns full file paths slice
|
|
func GetFullFilePathsInFolder(folder string) []string {
|
|
currentPath, err := os.Getwd()
|
|
if err != nil {
|
|
log.Fatal(err)
|
|
}
|
|
|
|
fullFolderPath := path.Join(currentPath, folder)
|
|
files, err := ioutil.ReadDir(fullFolderPath)
|
|
if err != nil {
|
|
log.Fatal(err)
|
|
}
|
|
|
|
var paths []string
|
|
|
|
for _, file := range files {
|
|
paths = append(paths, path.Join(fullFolderPath, file.Name()))
|
|
}
|
|
|
|
return paths
|
|
}
|
|
|
|
// Reads file contents by full path and returns string
|
|
func ReadFileText(fullFilePath string) string {
|
|
file, err := ioutil.ReadFile(fullFilePath)
|
|
if err != nil {
|
|
log.Fatal(err)
|
|
}
|
|
|
|
return string(file)
|
|
}
|