mirror of
https://github.com/yanislav-igonin/micrach
synced 2025-07-12 05:24:35 +03:00
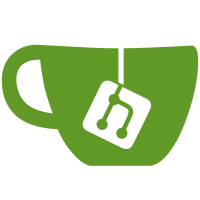
* feat: add geateway config * feat: add ping controller for check by gateway * feat: add new env vars for gateway * feat: update config * gateway request wip * feat: add auth header for gateway request * feat: separate gateway folder * update endpoint for gateway * add makefile * lint * swap png to svg * add url of board to request to gateway * change deployed path prefix * add env in compose * add body log on gateway connect
40 lines
926 B
Go
40 lines
926 B
Go
package gateway
|
|
|
|
import (
|
|
"bytes"
|
|
"encoding/json"
|
|
"io/ioutil"
|
|
"log"
|
|
"net/http"
|
|
|
|
Config "micrach/config"
|
|
)
|
|
|
|
// Make http request to gateway to tell the board id and description
|
|
func Connect() {
|
|
requestBody, _ := json.Marshal(map[string]string{
|
|
"id": Config.App.Gateway.BoardId,
|
|
"name": Config.App.Gateway.BoardDescription,
|
|
"url": Config.App.Gateway.Url,
|
|
})
|
|
url := Config.App.Gateway.Url + "/api/boards"
|
|
req, err := http.NewRequest("POST", url, bytes.NewBuffer(requestBody))
|
|
if err != nil {
|
|
log.Panicln(err)
|
|
}
|
|
req.Header.Set("Authorization", Config.App.Gateway.ApiKey)
|
|
req.Header.Set("Content-Type", "application/json")
|
|
client := &http.Client{}
|
|
resp, err := client.Do(req)
|
|
if err != nil {
|
|
log.Panicln(err)
|
|
}
|
|
//We Read the response body on the line below.
|
|
body, err := ioutil.ReadAll(resp.Body)
|
|
if err != nil {
|
|
log.Panicln(err)
|
|
}
|
|
log.Println(string(body))
|
|
log.Println("gateway - online")
|
|
}
|